I ensure you that by the end of this tutorial you are going to be the master of Python IF statement. This Python Programming tutorial is going to teach you many new things. So, sit back and enjoy. As this is going to be your ultimate guide for Python IF statement.
Execution Structure.
In order to understand what is a Python if statement we first need to know what are execution structures?
A logical process which tells the flow of execution of a program is know as the structure of execution. There are two types of structures available, and these are –
- Sequential structure, and
- Controlled structure.
Sequential Structure.
A structure where a set of statements execute in the order in which they are written is called Sequential Structure. For example
name = input ("Enter the name of the student: ") Tmarks = int (input ("Enter the total marks of the student:")) print("Congratulation! Student”, name , " Got - ", Tmarks, "marks.")
This program has three statements. And on execution all three of them will run one after another.
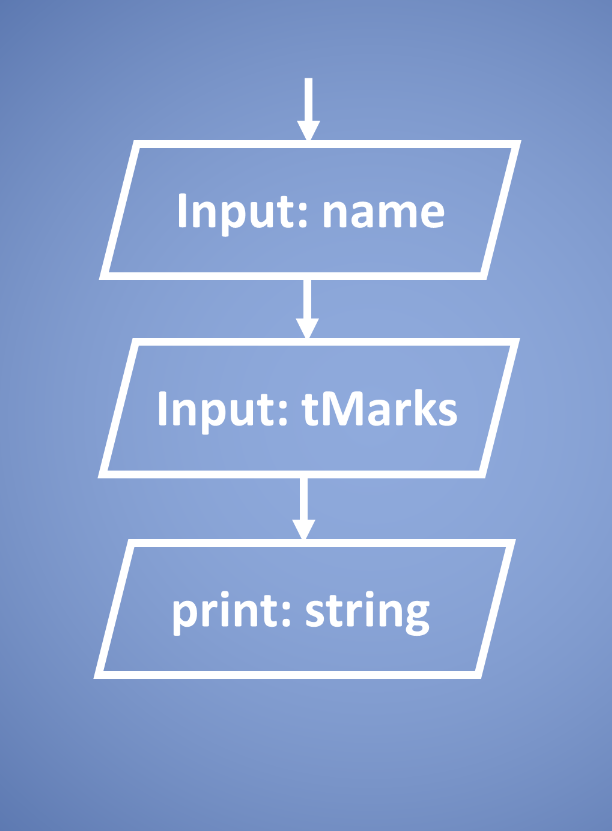
Controlled Structure.
A logical structure where we can control the flow of the execution of the program is called a controlled structure.
Also, there are two types of controlled structure, these are –
- Conditional Controlled and
- Looping
Python If statement is the best example of Conditional Control Structure, thus in this tutorial we will focus on it. Furthermore, we will talk about looping in future tutorials.
What is Python IF statement?
Not only in Python Programming but in any computer programming language, the best example of conditional control structure is ‘If Else’ statement.
Definition of Python If statement
Python ‘if statement’ is a bidirectional single alternative condition control decision structure.
I know the definition sounds a little technical. But, don’t worry just follow along & you will understand. In this blog, I have given the explanation of every technical word used in the definition.
The Syntax of Python IF statement.
The syntax of Python IF statement is very simple. Take a look at it—
if condition: block of statements
The statement starts with the keyword ‘ if ’. Followed by that we have ‘condition’. To illustrate, the condition is nothing but an expression which gets evaluated either as ‘true’ or as ‘false’. Meanwhile, right after the condition, we have a colon (:). Since in Python programming it is mandatory to put a colon right after writing the condition or expression in IF clause.
Let’s call this combination of the keyword ‘if’, the condition as well as the colon (:) – the if clause.
Right after our if clause we have a block of statements. Consequently, this block can contain either one or more than one executable statements.
Examples of Python If Statement.
Before, in the definition, we learnt that If statement is a conditional controlled decision structure. Now, let’s try to understand what that means through the help of an example.
Example 1: Python IF is a Conditional Controlled Decision Structure
Let’s say you want to write a code that will help your classmates to know whether they are promoted to the next semester or not.
Meanwhile, the promotion criteria decided by your college is the marks. Consequently, any student who has secured more than 45% of marks will be promoted to the next semester.
So, you have decided to use this information for your program.
min_marks_pct = 45 Total_marks = 1100 marks_obt = float(input("Enter Your Total Marks: ")) #Calculate the percentage secured by the student pct_obt = marks_obt/11 #If statement if pct_obt >= min_marks_pct: print("You Have Secured:", pct_obt , "%") print("Congratulations! You Are Promoted", end='\n\n') print("Thanks and Enjoy Your Day!")
This Python program is divided into three parts. Let me explain those to you –
Part 1: Line No. 1 to 3
In the first three lines we are declaring and initializing the Python Variables. Let’s see what are those variables?
min_marks_pct = 45 Total_marks = 1100 marks_obt = float(input("Enter Your Total Marks: "))
Suggested Reading: Python Tutorial 14 – Python Variables
Min_mark_pct: The first variable is the minimum marks percentage. In this variable we have stored 45 which is the minimum percentage required to get promoted into the next semester.
Total_marks: In the second variable we have stored 1100. Let’s suppose this is the total number of marks of all the exams.
Marks_obt: The third variable is the marks obtained. In this variable we are taking a float input from the user. Consequently, here the user has to enter the total marks he or she has secured.
Part 2: Line No. 6
In this part we are calculating the percentage obtained by the student using the values of variables from part 1. As a result, that calculated value of the percentage will get stored into the variable pct_obt
#Calculate the percentage secured by the student pct_obt = marks_obt/11
Part 3: Line No. 9 to 11
This is the main part of our program. Because here we are using the Python IF statement to make the decision.
#If statement if pct_obt >= min_marks_pct: print("You Have Secured:", pct_obt , "%") print("Congratulations! You Are Promoted", end='\n\n')
Firstly, what is that decision?
This Python if statement is helping us in deciding whether our classmates are promoted to the next semester or not. Since the Python IF statement is helping us in making the decision hence the name decision structure.
Now, let’s come to this Python If statement. As we know that an If statement has two parts. An If clause and the body. Let’s first check out the if clause.
In the if clause we are checking if the value stored in the variable pct_obt is greater than or equal to the value stored in the variable min_marks_pct. Furthermore, if this expression gets evaluated as true, then the body of this If statement will get executed. Otherwise, the control will skip the body and come out from the if statement.
Lastly the Python print statement.
If you see the program carefully then you will find out that right after the IF statement, we have an extra Python Print function call. This Python Print function call is completely outside the scope of the If statement. Thus, it will execute every time we run this program.
print("Thanks and Enjoy Your Day!")
Suggested Reading: Python Print Function.
Now that you have seen an example of the Python IF statement, understanding the definition of the if statement will be easier for you.
Python If – Why Conditional Controlled Structure?
As you can see, in this program the flow of execution is controlled by the condition used as the expression of the Python IF statement, hence the name condition control statement.
Python If – Why Decision Structure?
Above Python if statement is helping us in deciding whether our classmates are promoted to the next semester or not. Since the Python IF statement is helping us in making the decision hence the name decision structure.
Python if – A Single Alternative Structure.
Now you ask – In the definition you also said that the Python If statement is a single alternative structure. What do you mean by that?
In order to explain that, I need to draw a flow chart. Take a look at that here —
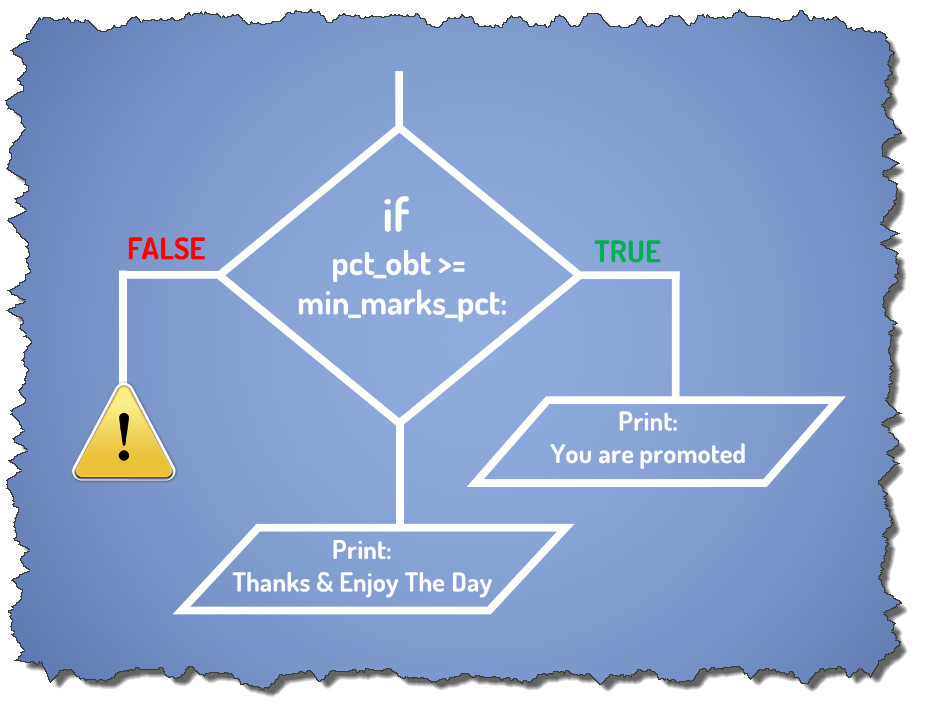
Here is the rough flow chart of the Python if statement which we just performed. As you can see in this chart that we only have one alternative path of execution. And that is when the if clause gets evaluated as true. Moreover, if the clause gets evaluated as false, then we have no path of execution for that.
Since Python if statement provides only one alternative path of execution hence we call it single alternative structure.
Why Conditional Controlled Decision Structure?
In a single alternative decision structure, a set of statements execute only if a certain condition exists. If the condition does not exist, then the action is not performed.
For example, in the above program, the students are getting informed that they are promoted only when the condition pct_obt >= min_marks_pct gets satisfied.
So that is the explanation of the definition that I gave to you earlier in this blog. Now let’s move on and learn how to overcome the shortcomings of a single alternative structure.
An if statement will work without the Else clause but there are very few chances when you will find yourself using a single alternative structure. Consequently, Dual alternative decision structure gives more flexibility and helps in making the code more user-friendly. Therefore, a dual alternative structure is preferable over the single alternative one. Let’s learn how to overcome the shortcomings of If statement in Python using the else clause.
But before that, if you are like me who learns better by watching a video then you can go ahead & check out the Python video tutorial that I’ve done on this topic.
And now back to the point…
Python If-Else Statement
The problem with the single alternative controlled structure is that – it is only good so long as the expression/condition of the if clause is evaluated as true. But, what if the condition is false. In such a situation we need a dual alternative structure. And that structure is Python If-Else Statement.
What is Python If-Else Statement?
Python If-Else statement is a dual alternative conditional control decision structure.
The Syntax of Python If-Else statement?
if condition: block of statements else: block of statement
A Python if-else statement executes one set of statements if the condition is true. And another set of instructions if the condition is false. Because of this,
we call a Python if-else statement a dual alternative structure.

Example of Python if-else statement.
min_marks_pct = 45 Total_marks = 1100 marks_obt = float(input("Enter Your Total Marks: ")) #Calculate the percentage secured by the student pct_obt = marks_obt/11 #If statement if pct_obt >= min_marks_pct: print("You Have Secured:", pct_obt , "%") print("Congratulations! You Are Promoted", end='\n\n') else: print("Only Those Students Securing Over 45% Marks Will Be Promoted") print("Your Aggregate % is:",pct_obt) print("Sorry! You Are Not Promoted.", end = '\n\n') print("Thanks and Enjoy Your Day!")
Here is the example of Python if-else statement. Except from the else block everything is pretty much the same. Now whenever a student scores less than 45% marks, he or she will not be kept in the dark. Instead he/she will be properly informed that he/she has not been promoted.
That is how we properly write a Python If-Else statement.
Python If – Precautions
There are a few precautions which you must take care of whenever you write a Python if statement. These are —
- Always put colons (:) after the if, elif and else keyword.
- Python is a case sensitive language so make sure to write the keywords if, elif and else in small letters.
- Indentation matters – In Python programming we use indentation to define the scope of an if or else clause. All the statements which you want to execute under the if clause or else clause should be indented towards the right-hand side. And by this I mean increasing the left margin by adding some blank spaces. Moreover you can do it simply by pressing the tab key.
Rules of indentation
- The indentation of the keywords like if, elif and else should be aligned. Meaning they all should be at the same level.
- Both, the if clause and else clause are followed by a set of statements. So, make sure the statements in the block are constantly indented.
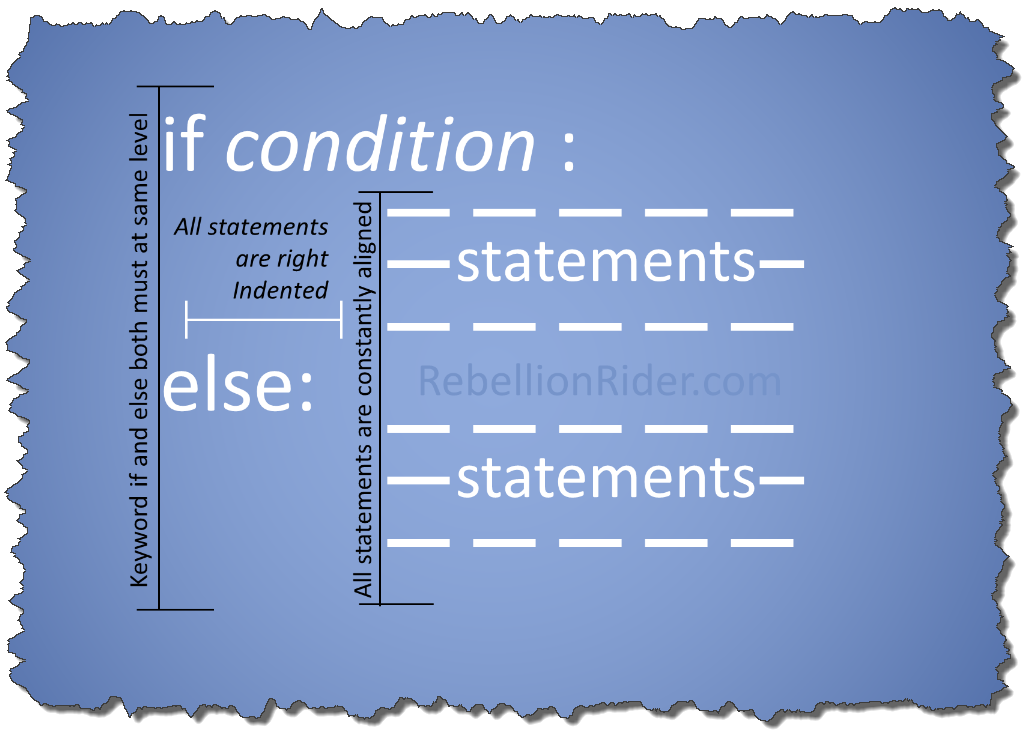
That is your complete guide on Python If statement. Stay tuned, as in the next Python tutorial we will learn how to further improve our code by solving its problems by using nested decision structure in Python Programming. You can also connect with me on my Facebook Page for more interesting content on Python.
Thanks for reading. Good Luck and Godspeed.