I bet what you are going to learn here is not something you must have known before. So, sit tight and let me show you the expert way to use Python Print Function.
The Print ( ) function is nothing new to Python Programming world. We have been using its curtailed version since the beginning of this series, but it’s much more than that. Therefore, in this tutorial, I will show you how to override the default settings of the Python Print function to use it to the fullest.
But first, let’s find out if the print is a statement or a function.
Python Print a statement or a function?
To begin with, the print was a statement but now it is a function. Consequently, the print used to be a statement in Python 2 but with the launch of Python 3, it became a function. Previously in Python 2 if you have to display some data then you just have to write a simple statement with the text or expression which you want to print. Like this
print “Python is Fun with RebellionRider”
But as I said, with the launch of Python 3 the print became a function.
Therefore, in Python 3, in order to display the data back to the user, you have to call the Python print in a proper function way. Where we have a function name along with parenthesis which are holding some arguments. Something like this
print (“Python is Fun with RebellionRider”)
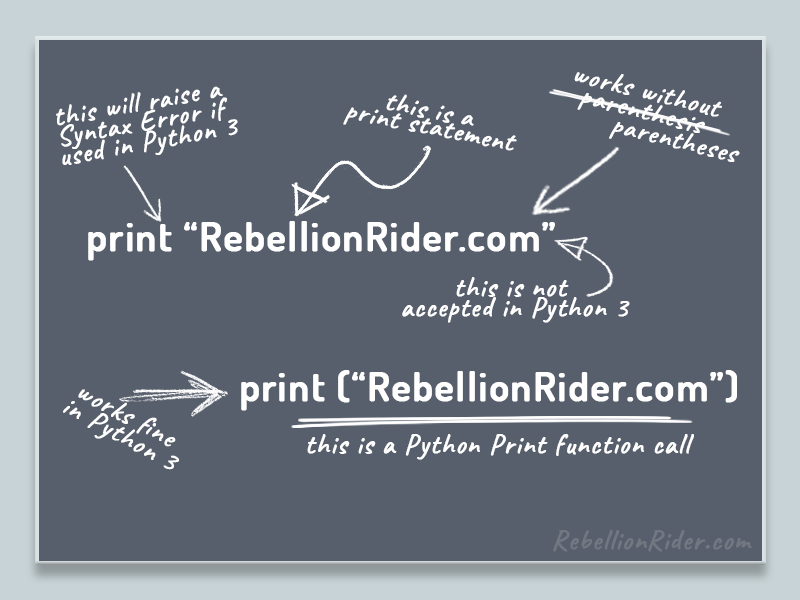
Info: Python 3 is not backwards compatible. Which means the print statement (The Python 2 version) is not acceptable in Python 3.
Meanwhile, are you waiting for the definition of Python Print function? I will give you the proper one right after discussing the syntax.
The Syntax of Python Print Function.
The Python Print function calls we have seen or written so far in this entire series are running pretty much on default settings. However, you can override these default settings and do a certain amount of formatting with your output. That is, if you know the syntax.
print(
object/s,
sep = ‘ ’,
end = ‘\n’,
file = sys.stdout,
flush = false
)
As I said earlier, The Print function is not as simple as it looks. This simple looking Python Print function takes five arguments. Let’s discuss each of them.
Object
Object is something you want to display back to the user on the standard output stream. It could be a string, an integer, value derived from an expression etc. Furthermore, you can use a single Print function call to show more than one object at once.
sep
‘sep’ is an acronym for the separator. This is the string which will get placed between two objects. The default value of ‘sep’ is set to blank space. The keyword ‘sep’ is case sensitive which is why you have to make sure that it must be written in all small letters.
end
‘end’ is again a string, which gets inserted at the end of the compiled text of print function call. By compiled text, I mean by the end result derived either after evaluating the expression or processing the objects like integer or string. Moreover, The default value of ‘end’ parameter is set on \n escapes sequence which is an indicator for the next line.
file
‘file’ parameter lets you specify your output stream. Consequently, using this parameter, you can set where your output will go. By default, this is set on the standard output stream, which in most cases is your computer screen. However, you can change it to a file. Then your output will get saved onto a file instead of being shown on your screen.
flush
‘flush’ is a Boolean parameter. It lets you decide if the output data is buffered or flushed. If you set it to true, then all the data of your output stream will get flushed. On the contrary, if it is set to false, then the data will get buffered. By default, it is set to false.
Are those parameters mandatory or optional?
All the parameters which we just discuss are optional. Which means if you want you can skip all those. In case you decide to skip any of the parameters then the Python interpreter will use their default values. For example
print(“This Python Tutorial is awesome”)
In this statement I have only used the first parameter of the function. Rest four parameters are missing. On execution, the Python interpreter will use the default values of all the four parameters which I have not used in the above Python ‘print’ function call.
What is Python Print Function?
Finally, it’s time for me to give you the proper definition.
The Print is an in-built function of Python library which displays the result which is derived from one or more ‘objects’ separated by ‘sep’ string and followed by the ‘end’ string to the ‘stream file’.
Based on the syntax, I think this is the best technical definition one can think of! But, if you have a better one, tell me in the comment section.
Examples of Python Print Function.
In this section I will show a few examples of Python Print Function which will help you in understanding the concept.
Print function with Object Parameter
In the first example we will explore the first parameter of the print function which is object. In fact, in Python world everything is object. All the data types of Python programming language are also objects.
print(“What’s up Internet”)
The above print( ) function call has one parameter which is a string object “What’s up Internet”. On execution it will be printed on your default output stream which in most cases is your computer screen.
In this function call we used only one object. But we can use single print function call to display more than one objects. Like this
print(“what’s up Internet”, “I am Manish”)
On execution you will see, both these objects printed on the screen separated by a blank space.
To take advantage of other parameters of the print function call make sure to separate all the objects using commas. If you want you can ditch the comma, there will be no problem until the object you are displaying is a string.
print(“what’s up Internet” “I am Manish”)
Consequently, Python interpreter will print both the objects on the screen but there will be no space between objects in the output.
Can we skip the comma if the objects are not strings?
Skipping comma will only work with the string objects. Contrarily, if the object is an integer or a mathematical expression, then that one missing comma will cause the Syntax Error. Like this
#This is a valid print function call print(5+5, 2*2)
The above print function call is a valid statement. Here I have python print function call containing two simple mathematical expressions. A comma separates both these expressions. On execution we will get a proper result. But if you delete the comma this Python Print function call will give you a syntax error.
#This statement will give you a syntax error print(5+5 2*2)
Watch the video tutorial to know why we get a syntax error on not separating the integer object by a comma.
Print function with sep Parameter
In the above example we saw that, when print function has more than one objects separated by comma then as a result we got both the strings printed and both are separated by a blank space. This is because the default value of ‘sep’ is set to blank space.
In addition if you want your objects to be separated by something other than a blank space then you can change the value of ‘sep’ parameter. For example
Let’s say I want to separate my objects by triplet of greater than sign (>>>) instead of a blank space.
print ("Python is fun", "But only with RebellionRider”, sep=“ >>> ”)
The above statement has two objects both are strings. On execution, the print function will print both the objects. However this time both will be separated by a string which we have specified in ‘sep’ parameter.
To change the separator. Firstly, you must put a comma after your objects then you have to write the keyword “sep”. Mind here the keyword “sep” is case sensitive. Followed by the keyword you have to specify your desired string which you want to place between the objects. This string must be enclosed inside a pair of colons. Consequently, it could be a single colon or a double colon.
Print function with end parameter
end- is again a string which is inserted at the end of the compiled text of Python print function call. Like ‘sep’, ‘end’ is also case sensitive. By default, it is set on \n escapes sequence which is an indicator for the next line.
To demonstrate how the end parameter actually works, I will write two print function calls.
print(8*3+6/3-8) print(“this is a string”)
On Execution, you will see that the output of both these print function call will be printed in separated lines rather than next to each other. This is because the default settings of the Python Print function is set to use ‘\n’ as ‘end’ parameter.
This parameter tells the Python interpreter what to do after printing the result of a print function call. You can override this default settings by changing the value of the said parameter.
Let’s say I don’t want the output of the second print statement printed in a new line. Rather I want it to print right next to the output of the first print statement.
print(8*3+6/3-8, end='\t') print(“this is a string”)
To achieve the result, I changed the default value of the ‘end’ parameter with the ‘\t’ in the first statement. On execution, the interpreter will not jump into the new line after printing the output of the first Python Print function call to print the result from second ‘print’ function call.
As the ‘file’ parameter is a concept in itself, thus we will discuss the remaining two parameters which are ‘file’ and ‘flush’ in the next tutorial.
What does the Python Print Function returns?
As all the parameters of the ‘print’ function are optional thus you are free to call it without any arguments. In case if you do so, then the print function will return ‘None’.
In Python ‘None’ is a data type which signifies ‘Null’ value. Mind here the ‘None’ data type implies to null values not zero.
You can take help of this simple code to find out what the Python Print function returns.
x = print() type(x)
The inbuilt function ‘type’ returns the data type of the object which is specified as its argument.
Write this code in your command prompt and see what it will return.
That’s it for this tutorial. Hope you enjoyed reading and learnt something new. In case if you have any doubts then feel free to drop me a message on my Facebook or Twitter. Thanks, and have a great day!